1. How to develop and debug a full authorized Polarion LiveDoc¶
Welcome to the capella2polarion notebook where local development and debugging of an FA Polarion LiveDoc is showcased. Full-authorized means, that we want to generate a live document as a whole without any interference in content from a human.
This notebook will show you the following:
How to load all current Polarion work-items into the PolarionWorker
How to load all current Polarion LiveDocs under a specified space
How to create and update Polarion LiveDocs under a specified space
Before we can interact with the REST API of Polarion we need to prepare our environment: Create a .env
file with the following values:
POLARION_PROJECT
POLARION_HOST
POLARION_PAT
MODEL_PATH
First the load the Capella model with capellambse:
[7]:
from capella2polarion.connectors import polarion_worker
from capella2polarion.converters import document_renderer, document_config
import dotenv
import os
import capellambse
import pathlib
dotenv.load_dotenv()
test_data_path = pathlib.Path("../../../tests/data")
model = capellambse.MelodyModel(os.environ.get(
"MODEL_PATH") or str(test_data_path / "model/Melody Model Test.aird")
)
worker = polarion_worker.CapellaPolarionWorker(
polarion_worker.PolarionWorkerParams(
os.environ.get("POLARION_PROJECT") or "",
os.environ.get("POLARION_HOST") or "",
os.environ.get("POLARION_PAT") or "",
delete_work_items=False,
)
)
1.1. How to load all current work items from the Polarion project into the worker:¶
[8]:
worker.load_polarion_work_item_map()
Now we load all current LiveDocs under a specific space. This is configured in the document config capella2polarion_document_config.yaml
:
[9]:
document_rendering_config_path = pathlib.Path("configs/capella2polarion_document_config.yaml.j2")
print(document_rendering_config_path.read_text(encoding="utf8"))
# Copyright DB InfraGO AG and contributors
# SPDX-License-Identifier: Apache-2.0
full_authority:
- template_directory: document_templates
template: test-pcd.html.j2
heading_numbering: True
work_item_layouts:
_C2P_componentExchange:
fields_at_start:
- id
fields_at_end:
- context_diagram
_C2P_physicalLink:
fields_at_start:
- id
_C2P_physicalActor:
fields_at_start:
- id
fields_at_end:
- context_diagram
_C2P_physicalComponentNode:
fields_at_start:
- id
fields_at_end:
- context_diagram
_C2P_physicalComponentBehavior:
fields_at_start:
- id
fields_at_end:
- context_diagram
_C2P_physicalFunction:
fields_at_start:
- id
fields_at_end:
- context_diagram
instances:
{%- for pc in model.search("PhysicalComponent") %}
- polarion_space: PC-Drafts
polarion_name: {{ pc.uuid }}
polarion_title: {{ pc.name | safe }}
params:
uuid: {{ pc.uuid }}
title: {{ pc.name | safe }}
kinds:
- SOFTWARE
- HARDWARE
{% endfor %}
As you can see we configured the work item layouts, i.e. work item representation in a live doc, for all work item types we expect from the template. The human should never modify the configuration because it will be overwritten by the service:
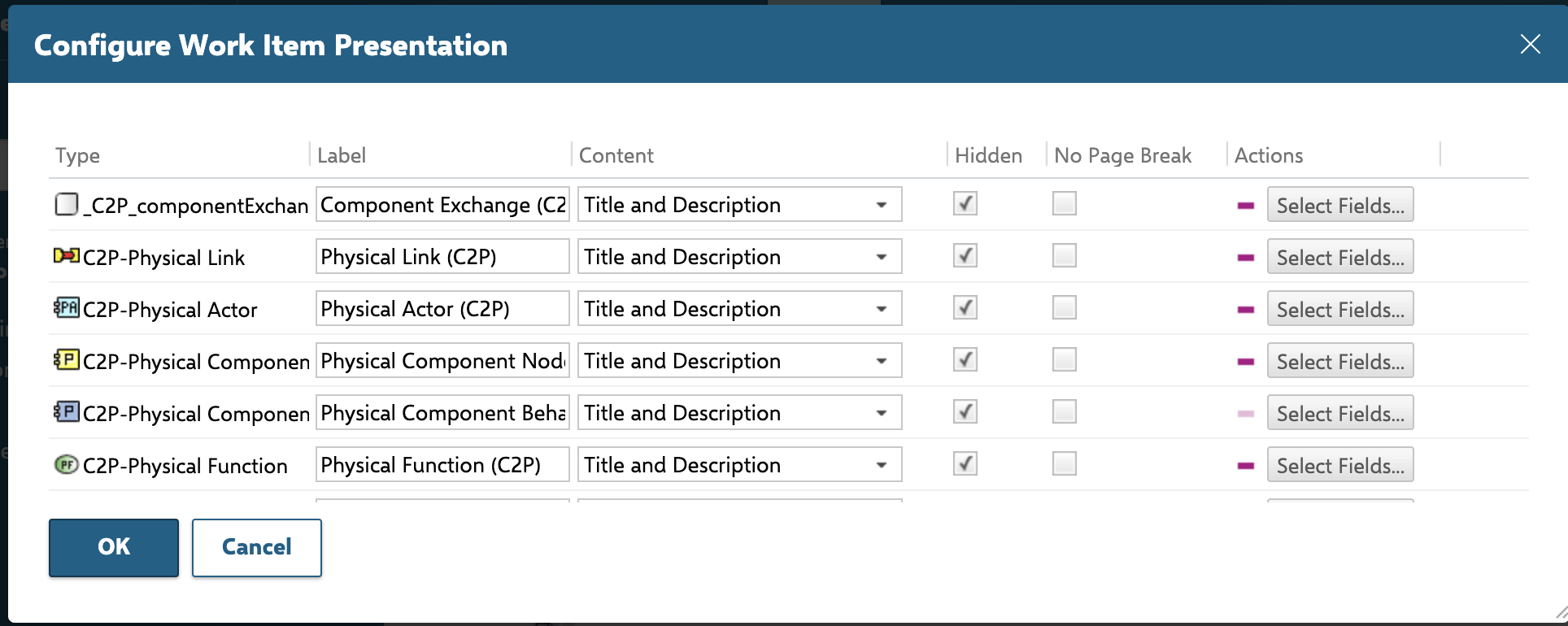
If you want to know more about the features and limitations, head into the documentation of the configuration for live doc rendering.
1.2. How to load all current Polarion LiveDocs under a specified space¶
We need a DocumentRenderer
and set it up with the following parameters:
[10]:
renderer = document_renderer.DocumentRenderer(
worker.polarion_data_repo,
model,
os.environ.get("POLARION_PROJECT") or "",
overwrite_heading_numbering=True,
overwrite_layouts=True,
)
with document_rendering_config_path.open("r", encoding="utf8") as file:
configs = document_config.read_config_file(file, model)
documents = worker.load_polarion_documents(configs.iterate_documents())
From the config file we can compute the documents (for each Physical Component one).
1.3. How to create and update Polarion LiveDocs under a specified space¶
[11]:
projects_document_data = renderer.render_documents(configs, documents)
for project, project_data in projects_document_data.items():
worker.create_documents(project_data.new_docs, project)
worker.update_documents(project_data.updated_docs, project)
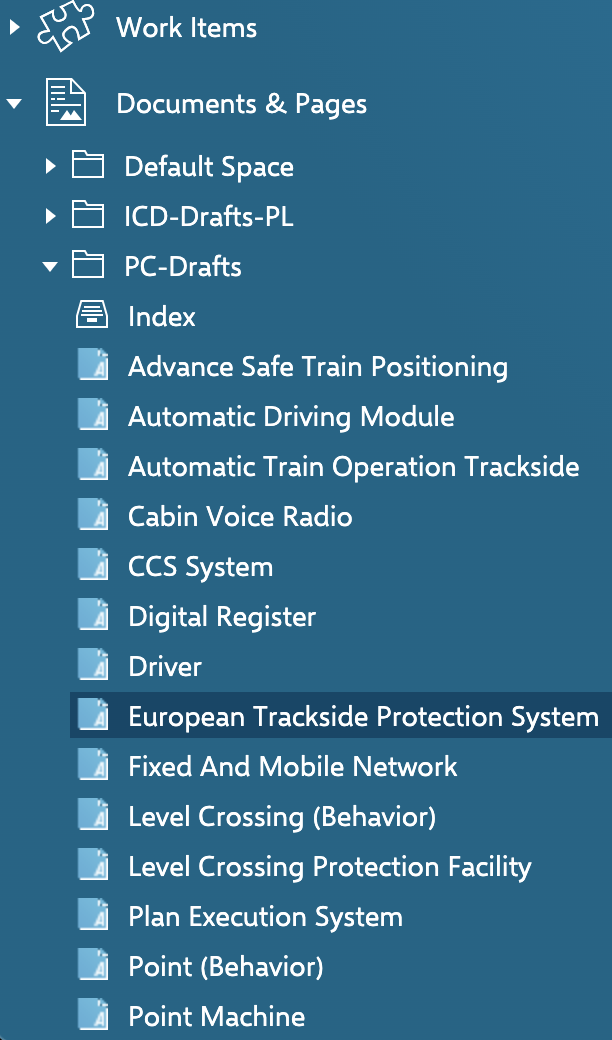